Defined in: <array>
Overview
template <typename T, std::size_t N>
class array
std::array
is a container that encapsulates fixed size arrays. It is a safer alternative to C-style arrays - T arr[N]
.
Example usage
The examples in this section are very simple ones. Navigate to examples section at the bottom for more.
- Create
- Fill and print
- Sort
- C++20
- C++17
- do C++17
- String literal
To create an array with an automatically deduced size or/and type,
we can use std::to_array
(since C++20).
Note: type and size deduction (at the same time) was possible since C++17.
#include <array>
int main() {
// type: std::array<int, 4>
auto ints = std::to_array({1, 5, 3, 7});
// type: std::array<float, 4>
auto floats = std::to_array<float>({1, 5, 3, 7});
// type: std::array<char, 6>
auto hello = std::to_array("Hello");
// this is different to std::array{"Hello"}, which creates std::array<char const*, 1>
}
Using CTAD
#include <array>
int main() {
std::array numbers{1, 2, 4, 8};
// Content: 1,2,4,8
// ❌ Error, cannot deduce size only (look C++20 to_array)
std::array<int> numbers{1, 2, 4, 8};
}
Unfortunately no deduction.
#include <array>
int main() {
std::array<int, 8> numbers = {1, 2, 4, 8};
// Content: 1,2,4,8,0,0,0,0
}
Note: this is only recommended if you don't have C++20, otherwise use std::to_array
.
#include <array>
int main() {
std::array<char, 6> str = { "Hello" };
// Content: 'H','e','l','l','o', <nul>
}
#include <iostream>
#include <array>
int main()
{
std::array<int, 4> playerScores{}; // initialize with zeros
for (int score : playerScores)
std::cout << score << ", ";
playerScores.fill(123);
playerScores.back() = 30;
std::cout << '\n';
for (int score : playerScores)
std::cout << score << ", ";
}
- C++20
- do C++20
Note: ranges
are available since C++20.
#include <array>
#include <algorithm>
int main() {
std::array<int, 4> numbers = {13, 2, 7, 4};
// Content: 13,2,7,4
std::ranges::sort(numbers);
// Content: 2,4,7,13
}
#include <array>
#include <algorithm>
int main() {
std::array<int, 4> numbers = {13, 2, 7, 4};
// Content: 13,2,7,4
std::sort(numbers.begin(), numbers.end());
// Content: 2,4,7,13
}
Memory
The elements of an array are stored contiguously in memory.
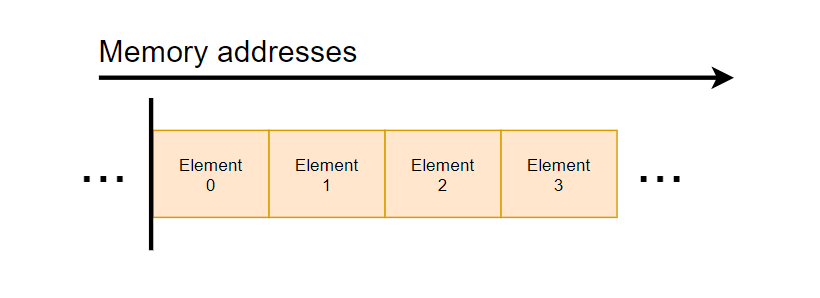
Technical details
Technical definition of an array
Zero-length array (N == 0
)
Named requirements
std::array
Defined in | array |
Template parameters
pub | T | Type of the elements |
pub | N | Number of elements (a compile-time constant) |
Type names
pub | value_type | T |
pub | size_type | std::size_t |
pub | difference_type | std::ptrdiff_t |
pub | reference | value_type& |
pub | const_reference | value_type const& |
pub | pointer | value_type* |
pub | const_pointer | value_type const* |
pub | iterator | LegacyRandomAccessIterator to value_type |
pub | const_iterator | LegacyRandomAccessIterator to value_type const |
pub | reverse_iterator | std::reverse_iterator<iterator> |
pub | const_reverse_iterator | std::reverse_iterator<const_iterator> |
Member functions
pub | (constructor) | Constructs an array following the rules of aggregate initialization. |
pub | (destructor) | Destroys every element of the array. |
pub | operator= | Overwrites every element of the array with the corresponding element of another array. |
Element access
pub | at | Accesses the specified element with bounds checking. |
pub | operator[] | Accesses the element. |
pub | front | Returns the first element. |
pub | back | Returns the last element. |
pub | data | Returns a pointer to the first element of the underlying array. |
Iterators
pub | begin cbegin | Returns an iterator/const_iterator to the beginning. |
pub | end cend | Returns an iterator/const_iterator to the end. |
pub | rbegin crbegin | Returns a reverse iterator/const_iterator to the beginning. |
pub | rend crend | Returns a reverse iterator/const_iterator to the end. |
Capacity
pub | empty | Returns true if the container is empty, otherwise false . |
pub | size | Returns the number of elements. |
pub | max_size | Returns the maximum possible number of elements. |
Operations
pub | fill | Fills the container with specified value. |
pub | swap | Swaps the contents. |
Non-member functions
pub | operator== operator!= operator< operator> operator<= operator>= operator<=> | Lexicographically compares the values in the array. |
pub | std::get (std::array) | Accesses an element of the array. |
pub | std::swap (std::array) | Specializes the std::swap algorithm. |
pub | std::to_array (C++20) | Creates a std::array object from a built-in array. |
Helper classes
pub | std::tuple_size<std::array> (C++11) | Obtains the size of an array. |
pub | std::tuple_element<std::array> (C++11) | Obtains the type of the elements of an array. |
Deduction guides (since C++17)
Click to expand
More examples
Using algorithms
Find minimal value (C++20)
#include <iostream>
#include <array>
#include <algorithm>
int main()
{
namespace rg = std::ranges;
auto numbers = std::to_array({ 12, 3, 18, 9});
// Find minimal element
auto minIt = rg::min_element(numbers);
// obtain value:
auto value = *minIt;
// find position:
auto index = std::distance(numbers.begin(), minIt);
std::cout << "Min: " << value
<< ", at index: " << index
<< std::endl;
}
Result
Min: 3, at index: 1
Advanced
Using array as a temporary buffer
#include <fstream>
#include <array>
#include <string>
std::string readFile(std::istream& file)
{
constexpr size_t BUFFER_SIZE = 16 * 1024; // 16 KB
constexpr size_t RESERVE_SIZE = 1 * 1024 * 1024; // 1 MB
std::string result;
result.reserve(RESERVE_SIZE); // 1 MB
std::array<char, BUFFER_SIZE> buf;
while(file.read(buf.data(), buf.size()))
result.append(buf.data(), buf.data() + file.gcount());
result.append(buf.data(), buf.data() + file.gcount());
return result;
}
// Example usage:
int main()
{
std::ifstream file("hello.txt");
if (file.is_open())
{
auto fileContents = readFile(file);
// ...
}
}
Concat two arrays (C++20)
#include <iostream>
#include <array>
#include <algorithm>
template <typename T, size_t N1, size_t N2>
auto concat(
std::array<T, N1> const& lhs,
std::array<T, N2> const& rhs
)
{
namespace rg = std::ranges;
std::array<T, N1 + N2> result;
rg::copy(lhs, result.begin());
rg::copy_backward(rhs, result.end());
return result;
}
int main()
{
auto left = std::to_array({1, 2, 3});
auto right = std::to_array({4, 5, 6});
auto both = concat(left, right);
for (int elem : both)
std::cout << elem << ' ';
}
Result
1 2 3 4 5 6
This article originates from this CppReference page. It was likely altered for improvements or editors' preference. Click "Edit this page" to see all changes made to this document.
Hover to see the original license.
Hover to see the original license.